Please select or create new application project once you arrived at the credential page, once you've done, the page will automatically be redirected to credential page. In this page, you have to navigate to Overview page. You will found the link at the left navigation. Choose and activate the Blogger API in Social APIs. Once it enabled, the API is ready to use.
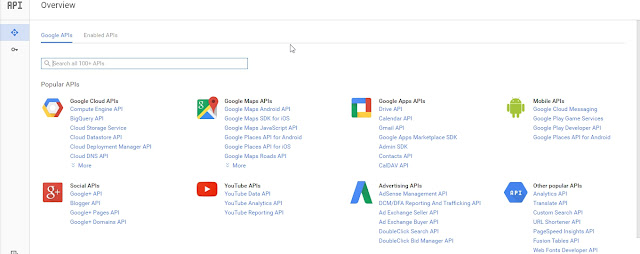
To fetch data we can use the $http service provided by AngularJS. It simple like:
app.controller('Blog', function($scope, $http, $routeParams) { $http.get("https://www.googleapis.com/blogger/v3/blogs/3383941000974617080/posts?key=YOUR_API_KEY") .then(function(res) { $scope.blog = res.data.items; }); });
Please change YOUR_API_KEY with your API key of course. :) I use the controller called 'Blog' to send http request. The response will be in JSON format. Like:
{ "kind": "blogger#postList", "nextPageToken": "CgkIChiAkceVjiYQ0b2SAQ", "prevPageToken": "CgkIChDBwrK3mCYQ0b2SAQ", "items": [ { "kind": "blogger#post", "id": "7706273476706534553", "blog": { "id": "2399953" }, "published": "2011-08-01T19:58:00.000Z", "updated": "2011-08-01T19:58:51.947Z", "url": "http://buzz.blogger.com/2011/08/latest-updates-august-1st.html", "selfLink": "https://www.googleapis.com/blogger/v3/blogs/2399953/posts/7706273476706534553", "title": "Latest updates, August 1st", "content": "elided for readability", "author": { "id": "401465483996", "displayName": "Brett Wiltshire", "url": "http://www.blogger.com/profile/01430672582309320414", "image": { "url": "http://4.bp.blogspot.com/_YA50adQ-7vQ/S1gfR_6ufpI/AAAAAAAAAAk/1ErJGgRWZDg/S45/brett.png" } }, "replies": { "totalItems": "0", "selfLink": "https://www.googleapis.com/blogger/v3/blogs/2399953/posts/7706273476706534553/comments" } }, { "kind": "blogger#post", "id": "6069922188027612413", elided for readability } ] }
So it so easy to display the data like title and content:
<div ng-repeat x in blog>{{x.title}}</div>
Or with filter like:
<div ng-bind-html="x.content | limitTo : 600 : 0"></div>
Please visit: http://makmalf.com/#/blog
No comments:
Post a Comment